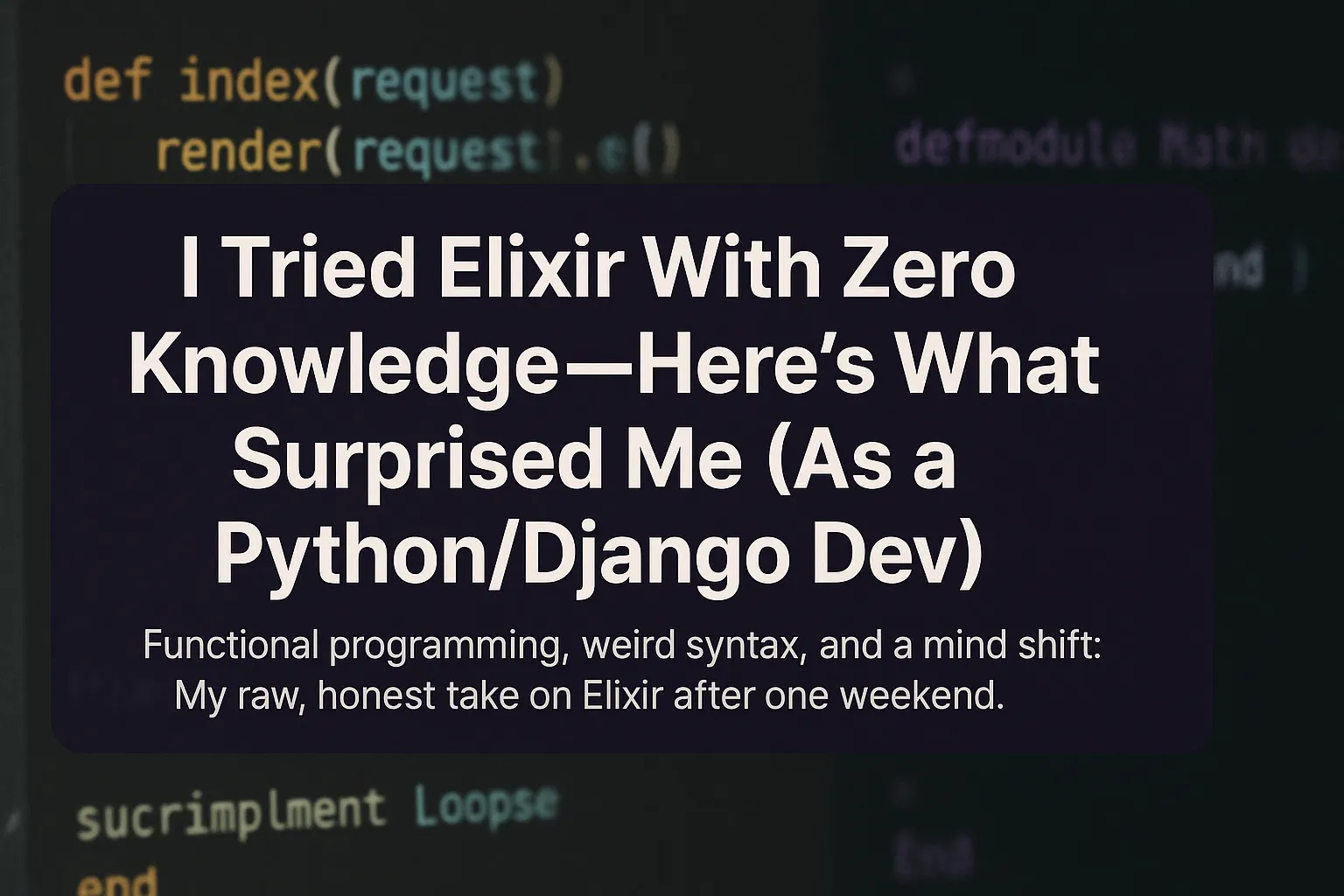
I Tried Elixir With Zero Knowledge — Here’s What Surprised Me
Why Elixir?
“I kept hearing about Elixir… so I finally decided to dive in.”
Let me be brutally honest — I didn’t start learning Elixir because I had some divine revelation about functional programming. I started because I kept seeing people in tech forums act like Elixir was the holy grail of backend development. You know the type: the ones who say, “Once you understand the BEAM, you’ll never go back.” Cool, bro. I barely understand the microwave.
But as a Python and Django developer, I’m always curious about what might make me more efficient, scalable, and, dare I say, future-proof. And Elixir just kept popping up in all the right conversations: real-time apps, fault-tolerant systems, massive concurrency, and something called the BEAM virtual machine that apparently laughs in the face of server crashes.
So, I figured: screw it. Let’s see what all the noise is about.
And wow — Elixir is weird. Not in a bad way, but in the same way that chess is weird the first time you play it. It’s not OOP. It’s not imperative. It’s functional, which means everything is immutable and side-effect free. Translation? No more sneaky variables changing behind your back. In theory, it forces cleaner code. In reality, it forces you to rethink everything you know about programming — or rage-quit and go back to JavaScript.
But here’s what really got me: Elixir is built for scale from the ground up. While most languages treat concurrency as a bolted-on afterthought, Elixir treats it like a first-class citizen. Thanks to the BEAM, Elixir can spawn literally millions of lightweight processes without breaking a sweat. Meanwhile, your Node.js app panics if a single promise misbehaves.
Elixir was designed for fault-tolerance — think telecom-level reliability. That’s not hype; that’s inheritance. It’s built on Erlang, which powers systems where “five nines” uptime isn’t impressive — it’s expected. And suddenly, I started wondering: Why aren’t more people using this thing?
Well, that’s what this post is here to explore.
If you’ve ever wondered what it’s like to dip your toes into Elixir as a complete outsider, buckle up. I’m documenting my journey — no sugar-coating, no gatekeeping, and absolutely no hype without receipts.
First Impressions
Elixir doesn’t try to look like other languages — and it sure as hell doesn’t care if you’re uncomfortable. The syntax hit me like a brain freeze from an unfamiliar Slurpee flavor. It’s clean, yeah, but also alien. No semicolons. No traditional loops. And defmodule
? That’s not a boss in Elden Ring, it’s how you define modules. Cute.
Instead of the usual Python-style readability where everything feels like a storybook, Elixir’s syntax feels like reading a poem written by a mathematician — precise, elegant, but you need a second read to get the punchline. It’s deceptively simple but subtly powerful. Think of it like writing music: once you stop thinking like a JavaScript dev and start thinking in patterns and flows, it starts to sing.
Then there’s the tooling.
mix
is Elixir’s answer to life, the universe, and everything. It’s the one-stop CLI for creating projects, managing dependencies, running tests, and basically making you feel like a wizard. Python devs, remember when you first found out about pipenv
or poetry
? Multiply that joy by ten, subtract the config headaches, and you’re still not at Elixir’s level of bliss.
And then there’s iex
— the interactive shell. If Python’s REPL is a helpful assistant, iex
is a fully loaded command center with god-tier debugging features. You can compile modules on the fly, inspect variables with h
, and even run live code in your app — without restarting it. When was the last time Django let you do that without diving into some obscure stack overflow thread from 2013?
Speaking of Django… let’s talk Phoenix.
Phoenix is Elixir’s web framework, and it's what happens when someone looks at Django and says, “Cool idea. Now let’s actually make it real-time, fault-tolerant, and fast.” It borrows the good parts of Rails and Django but throws in native support for things like LiveView, which lets you build interactive apps with zero JavaScript. Read that again. No JavaScript. Still getting a little misty-eyed just thinking about it.
Phoenix feels like what Django wants to be when it grows up — leaner, faster, and built for apps that don’t just scale, but thrive under pressure. If Django is a classic pickup truck that’ll never die, Phoenix is a Tesla running on caffeine and cold logic.
But no first impression would be complete without a moment of pure confusion. Enter: Pattern Matching.
At first, I thought pattern matching was just fancy destructuring. Nope. It’s a full-blown paradigm shift. You’re not just assigning values; you're asserting structure. Functions don’t use conditionals as much as they use patterns. Want a different outcome for a different input? Write a new function clause. It’s like switch statements on steroids — except cleaner, more expressive, and occasionally headache-inducing until it finally clicks. Then it's like Neo seeing the Matrix.
To summarize: Elixir isn’t just a new language. It’s a whole new mental model. And that’s either exciting or terrifying, depending on how married you are to your current stack.
What Confused Me
If Elixir were a person, it’d be that eccentric genius at the party who refuses to use a fork, eats cake with a spoon, and somehow pulls it off. The logic makes sense eventually, but only after you throw out half the mental habits you’ve collected from other languages. Here’s what twisted my brain the most.
Immutable Variables
This one hit hard. Coming from Python and JavaScript, I’m used to slinging variables around like I'm bartending at a startup — assign, reassign, mutate, profit.
But Elixir was like:
"Nah, fam. Variables don’t change. Ever."
In Elixir, everything is immutable. When you write:
x = 5
x = x + 1
You’re not updating x
. You’re rebinding it. Elixir doesn’t mutate values — it just gives you new ones. Which is cool conceptually... until you try to write anything that loops, mutates, or tracks a changing state, and suddenly you're sitting there like:
“Where’s my
while
loop?!”
This brings us to the second kick in the cognitive teeth:
Recursion Over Loops
No for
, no while
, no do...until
. Just recursion. Pure, beautiful, terrifying recursion.
So instead of this (in Python):
for i in range(5):
print(i)
You do this in Elixir:
defmodule Loop do
def print_nums(0), do: :ok
def print_nums(n) do
IO.puts(n)
print_nums(n - 1)
end
end
Loop.print_nums(5)
At first, this felt like building IKEA furniture with boxing gloves. But after a few reps, recursion started to feel more natural — like learning to write with your non-dominant hand, and realizing it might actually have better penmanship.
Plus, recursion and immutability together? Chef’s kiss. You start writing code that’s predictable, safe, and weirdly satisfying.
The Pipe Operator (|>
) – Weird at First, Then Kinda Cool
Elixir’s pipe operator is either the greatest gift to code readability or the first sign that you’re joining a cult.
But a useful one.
It lets you chain functions together in a readable, top-to-bottom way:
"hello"
|> String.upcase()
|> String.reverse()
|> IO.puts()
This feels very different from nesting functions like a deranged Russian doll:
IO.puts(String.reverse(String.upcase("hello")))
At first, |>
felt like syntactic sugar for people who hate parentheses. But once I started writing more functional code, it clicked. It's not just cleaner — it’s how Elixir flows. You’re literally piping the output of one function into the next, like composing a song.
Also, it makes your code read like instructions, not code. That’s powerful.
In short: Elixir doesn’t just confuse you — it reprograms you. Every “WTF” moment is followed by an “Aha!” And that slow crawl toward enlightenment is half the thrill.
What I Liked
Despite Elixir’s learning curve occasionally feeling like being slapped with a math textbook, there were a few things that genuinely made me stop, squint at my screen, and say out loud, “Okay… that’s actually pretty sick.”
Concurrency Made Simple (Hello, BEAM!)
First off — let’s talk about the BEAM. Not a laser. Not a spaceship part. But Elixir’s virtual machine — the heart of why Elixir scales like an absolute beast.
I didn’t fully get what “concurrency” meant in the brochure. You hear it in every Elixir post like it's a magic word, usually thrown in with phrases like “soft real-time systems” or “millions of lightweight processes.” But the moment I spun up a thousand concurrent processes without my laptop wheezing like it just ran a marathon — it hit me.
for _ <- 1..1000 do
spawn(fn -> IO.puts("Running async task") end)
end
Boom. No threads. No locks. No tears. Just lightweight, isolated processes, all chillin’ thanks to the BEAM’s scheduling wizardry.
This is where Python and Node quietly pack up their sleeping bags and go home. Elixir’s approach to concurrency isn’t just powerful — it’s damn approachable. You don’t need to study operating systems to get started. You just spawn stuff and let the BEAM handle it like a responsible adult.
Clean, Readable Functions
Elixir’s syntax may have confused me at first, but once I settled in, I realized something: this language reads like a conversation. The functions are clean, declarative, and almost poetic in their layout.
def greet(name) do
"Hello, #{name}!"
end
No fuss. No ceremony. Just direct, expressive code. Pattern matching lets you write multiple function heads for different scenarios, so you can replace ugly if-else
ladders with beautiful, branching logic that’s crystal clear.
def handle_input("yes"), do: "You agreed."
def handle_input("no"), do: "You declined."
def handle_input(_), do: "Invalid input."
That’s not just cleaner — it’s self-documenting. Your logic becomes your documentation, which is the holy grail of maintainability.
Elixir's Error Messages (Surprisingly Friendly)
Look, we’ve all been scarred by error messages. Python sometimes gives you a wall of traceback hell. Java throws stack traces that feel like legal contracts. JavaScript? Let’s not even talk about the lies that come from undefined is not a function
.
But Elixir? Elixir feels like it wants to help.
** (MatchError) no match of right hand side value: 42
It’s direct. It points to exactly where things went wrong. And the tone is oddly respectful. Like, “Hey, you probably meant to match something else. No judgment. We’ve all been there.”
Even compiler warnings in Elixir don’t feel like it’s yelling at you. They’re like gentle nudges from a kind mentor. “Hey, that variable isn’t being used. You might want to double-check.”
As someone who’s used to fighting their codebase for clarity, this level of developer empathy? Chef’s kiss. Debugging becomes a conversation, not a cage match.
So yeah — Elixir is weird, wild, and wonderful. Concurrency that doesn’t suck, functions that read like poetry, and error messages that feel like they’re written by a therapist. It’s a whole vibe.
How I’d Learn It Properly (If I Continue)
After surviving my first encounter with Elixir — dodging recursion-induced headaches, coming to terms with immutable everything, and realizing the BEAM isn’t a meme — I started thinking: Okay... if I were to really get serious about this, how would I learn it properly?
Because truth be told, Elixir doesn’t hold your hand. It throws you into the functional deep end and watches to see if you float. But if you know where to look, there are floaties — and some of them are pretty damn good.
ElixirSchool – The No-Nonsense Crash Course
ElixirSchool is like that chill professor who skips the 300-slide PowerPoint and just says, “Alright, here’s how this actually works.”
It’s clean, structured, and hits you with practical examples without bloating everything with 15 paragraphs of backstory. I especially appreciated how it explained concepts like pattern matching and supervisors without making me feel like I needed a PhD in computer science.
If you're looking for free, digestible, and straight-to-the-point — this is your dojo.
Joy of Elixir – Wholesome as Hell
Joy of Elixir is like if Bob Ross decided to teach functional programming. It’s written for absolute beginners, and instead of throwing concurrency and OTP in your face, it eases you in gently.
No scary words. No assumptions. Just Elixir, explained in a way that makes you feel like you’re reading a story, not a spec doc. It’s ideal if you want to understand the why behind Elixir, not just the how.
Honestly, I wish more languages had a “Joy of [Insert Language]” version. We’d all be a little saner.
What I’d Build – Something Phoenix-Flavored
Let’s be real — if I’m going to commit to Elixir, it won’t be for CLI scripts or some cute recursion games. It’ll be because Phoenix is a monster when it comes to performance.
That said, my first Phoenix project wouldn’t be another boring to-do list. I’d want to build something real — maybe a realtime voting app that updates live without the need for page reloads. Something that actually flexes Phoenix’s WebSocket-powered LiveView muscles and puts Django to shame with its real-time feel.
Imagine this:
- A live dashboard for audience polling
- Votes updating in real time
- Sessions expiring automatically
- Minimal JavaScript
That’s not just a tutorial project — that’s a legit portfolio piece. And the more I think about it, the more I realize... Elixir’s not just for hipster devs or distributed-systems nerds. It’s for builders who want scale, speed, and sanity, without selling their soul to Kubernetes.
So if I do go deeper into Elixir — and I just might — I’ll do it right. With the right tools, the right mindset, and the right project to push me. Because once you get past the functional culture shock, Elixir doesn’t just click.
It sings.
Final Thoughts
So after fumbling my way through Elixir’s syntax, getting brain-slapped by recursion, and falling slightly in love with the BEAM — the big question is:
Would I use Elixir in production?
Short answer? Yeah. If the project fits, absolutely.
Long answer? Yes, but not everything needs a concurrency powerhouse running on Erlang steroids.
If I were building the next Twitter, Discord, or some real-time financial dashboard that needs to juggle a crap-ton of users without catching fire, I wouldn’t even blink — I’d reach for Phoenix and Elixir.
It’s fast. It’s fault-tolerant. It scales horizontally like it’s on a mission. That whole “let it crash” philosophy actually works in practice — the language is designed to survive chaos.
But for your average CRUD-heavy admin dashboard or basic blog site? I’d probably still lean on Django or Laravel — not because Elixir can’t do it, but because it’s a bit like using a Ferrari to deliver pizza. You’ll impress people, but it’s overkill and unnecessarily complex for the job.
Who Should Look Into Elixir?
- Backend devs tired of writing async boilerplate in Node.js
- Pythonistas who secretly love clean code and want better concurrency
- Startups building real-time apps (chat, analytics, multiplayer, etc.)
- SaaS engineers who care about uptime and scaling without devops trauma
- Anyone who wants to understand functional programming in a way that makes sense
But let me warn you: Elixir will ruin other languages for you. You’ll start asking why every language doesn’t have pattern matching or built-in supervisors. You’ll see null
and flinch. You’ll dream of pipes.
Elixir doesn’t just teach you a new syntax. It rewires how you think about software.
“Will I Keep Learning It?” – Let’s Be Real
Honestly? Probably yes… but casually.
I’m not about to throw my current stack in the trash and move to Elixir Island full-time. But this isn’t a fling either.
I’ll keep exploring it on weekends, probably build that voting app I mentioned, and slowly pick up more OTP concepts. If a client project ever calls for massive concurrency or reliability on a Netflix level, Elixir's getting the first phone call. Until then, it’ll sit in my toolbelt, polished and ready, whispering:
“When you’re ready to build something powerful, I’m here.”
Elixir’s not for everyone. But for the right minds? It’s not just a language — it’s a revelation.
And if you’re a die-hard OOP dev who thinks classes are the One True Way? You’re exactly the kind of person who needs to try Elixir. Triggered? Good. Go learn something.
Comments