Building an Interactive Badge Scanner System with Python Text-to-Speech
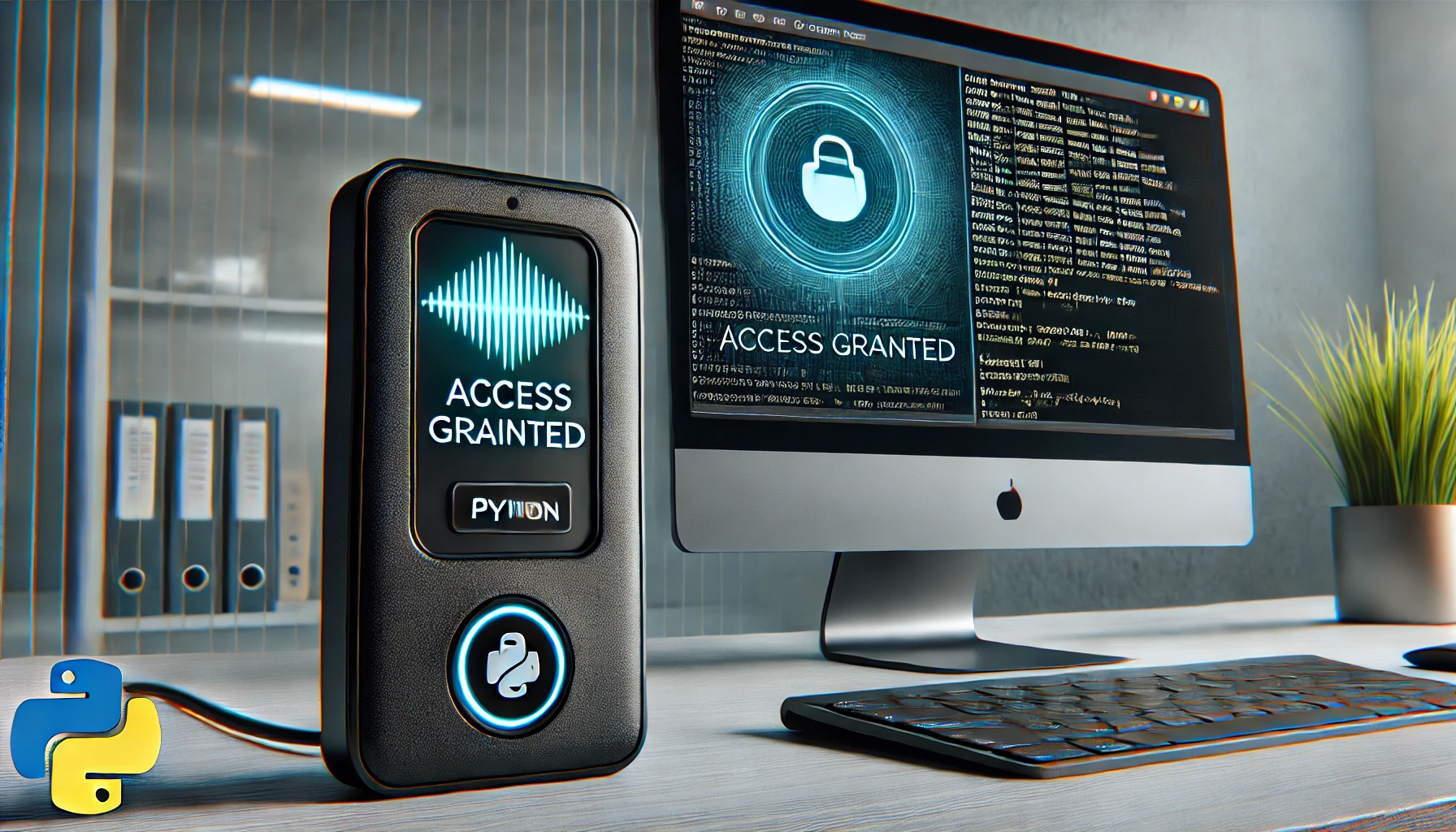
Access control systems play a crucial role in security and workforce management. In this tutorial, we'll build an interactive badge scanner system in Python that provides real-time audio feedback using text-to-speech (TTS). This system is ideal for offices, events, or any environment requiring badge-based authentication.
Why Build a Badge Scanner System?
- Automated Access Control – Streamline entry and exit processes.
- Audio Feedback – Get immediate spoken responses for badge scans.
- Real-Time Badge Validation – Ensure only valid badge holders are granted access.
- Scalability – Can be extended with database storage, time-based restrictions, and logging.
Prerequisites
To follow along, ensure you have:
- Python 3.x installed on your system.
- Familiarity with basic Python programming and package management using
pip
.
Required Packages
Install the necessary dependencies:
pip install pyttsx3 keyboard
These libraries enable text-to-speech output (pyttsx3
) and keyboard event handling (keyboard
).
Step 1: Setting Up Voice Notifications
We'll create a VoiceNotifier
class to handle spoken feedback:
import pyttsx3
class VoiceNotifier:
def __init__(self):
self.engine = pyttsx3.init()
def speak(self, message):
self.engine.say(message)
self.engine.runAndWait()
def access_granted(self):
self.speak("Access granted")
def exit_granted(self):
self.speak("Exit granted")
def access_denied(self):
self.speak("Access denied")
def badge_exists(self):
self.speak("Badge code already exists")
def badge_added(self):
self.speak("Badge code added")
The VoiceNotifier
class ensures smooth text-to-speech responses for all system events.
Step 2: Implementing the Badge Management System
Next, we create a BadgeManager
class to handle badge validation and scanning:
class BadgeManager:
def __init__(self):
self.voice = VoiceNotifier()
self.valid_badges = set(range(100000)) # Badge range: 00000-99999
self.active_badges = set()
def validate_badge(self, badge_code):
try:
return 0 <= int(badge_code) <= 99999
except ValueError:
return False
def scan_badge(self, badge_code):
if not self.validate_badge(badge_code):
self.voice.access_denied()
return False
badge_code = int(badge_code)
if badge_code in self.active_badges:
self.active_badges.remove(badge_code)
self.voice.exit_granted()
else:
self.active_badges.add(badge_code)
self.voice.access_granted()
return True
def add_badge(self, badge_code):
if not self.validate_badge(badge_code):
print("Invalid badge code format")
return False
badge_code = int(badge_code)
if badge_code in self.valid_badges:
self.voice.badge_exists()
return False
self.valid_badges.add(badge_code)
self.voice.badge_added()
return True
def get_active_badges(self):
return sorted(self.active_badges)
This class manages badge validation, tracks active badges, and prevents duplicate badge entries.
Step 3: Creating an Interactive Interface
We implement a keyboard-driven interface for scanning and adding badges:
import keyboard
class BadgeScanner:
def __init__(self):
self.badge_manager = BadgeManager()
def start(self):
print("Badge Scanner System")
print("Press 's' to scan a badge")
print("Press 'a' to add a new badge")
print("Press 'ESC' to exit")
keyboard.on_press_key("s", lambda _: self.scan_mode())
keyboard.on_press_key("a", lambda _: self.add_mode())
keyboard.wait("esc")
def scan_mode(self):
badge = input("Scan badge: ")
self.badge_manager.scan_badge(badge)
def add_mode(self):
badge = input("Enter new badge code: ")
self.badge_manager.add_badge(badge)
if __name__ == "__main__":
scanner = BadgeScanner()
scanner.start()
This script provides a user-friendly way to interact with the badge system via keyboard shortcuts.
How It Works
- Press 's' to scan a badge.
- Press 'a' to add a new badge.
- Press 'ESC' to exit.
Features & Benefits
- Instant Voice Feedback – No need to check screens; the system speaks the results.
- Badge Number Validation – Prevents invalid badge codes.
- Dual-Purpose Badge Scanning – Handles both entry and exit with a single scan.
- Expandable – Can be integrated with hardware scanners, databases, and logging.
Get the Complete Script
Want to skip the setup and get a ready-to-use script? The full Badge Scanner System is available for download at Brand Nova. Click the link below to access the product:
👉 Get the Badge Scanner System on Brand Nova
Using the Enhanced Script (Available on Brand Nova)
What’s New in the Enhanced Version?
🚀 Expanded Features:
- Dynamic badge registration 📋
- Check-in & check-out tracking 🔄
- More polished voice feedback 🎤
- Support for large badge databases 🏛️
How to Use the Enhanced Version
- Download the script from Brand Nova 💾
Install dependencies:
pip install -r requirements.txt
Run the script:
python run.py
- Follow the on-screen instructions to register and scan badges!
Future Enhancements
This system can be extended in several ways:
- Database Integration – Store badge data permanently.
- Logging System – Keep track of badge scans.
- GUI Interface – Replace CLI with a user-friendly dashboard.
- Hardware Support – Connect physical badge scanners.
Conclusion
This Python-based badge scanner system offers a practical, easy-to-use solution for access control. By integrating text-to-speech feedback, it enhances user experience and usability. Whether you're managing workplace access or tracking event attendees, this script provides a solid foundation for further customization.